Writing clean code in PHP is very important. It makes your code more readable and easier to understand for others. We’ll outline 5 tips to enhance your code, so you can write cleaner and more understandable code.
1. Give your variables proper names
When writing code you’ll be creating a lot of variables. That’s why it’s important to give them proper names, so you can easily see what a variable contains.
For example:
// Bad naming, d is not meaningful
$d = new \DateTime('now');
// Good naming
$currentDate = new \DateTime('now');
Code language: PHP (php)
The variable name in the first example doesn’t tell you anything about its contents, whereas $currentDate is immediately obvious.
2. Use constants instead of hardcoded strings or numbers
Sometimes you’ll need to use a hardcoded string in your code, for example when you want to set a role on your user object. It’s better to create a constant for that string and then reference that constant in the places you need to use that string.
For example:
// Bad
$user->setRole('ROLE_ADMINISTRATOR');
// Good
class User {
public const ROLE_ADMINISTRATOR = 'ROLE_ADMINISTRATOR';
public const ROLE_USER = 'ROLE_USER';
}
$user->setRole(User::ROLE_ADMINISTRATOR);
Code language: PHP (php)
In the example above we set a role on a User
object. In the bad example we need to add the ROLE_ADMINISTRATOR
string everywhere. This can lead to strange and hard to debug bugs. Using a constant makes sure you’ll get an error from PHP when you made a typo.
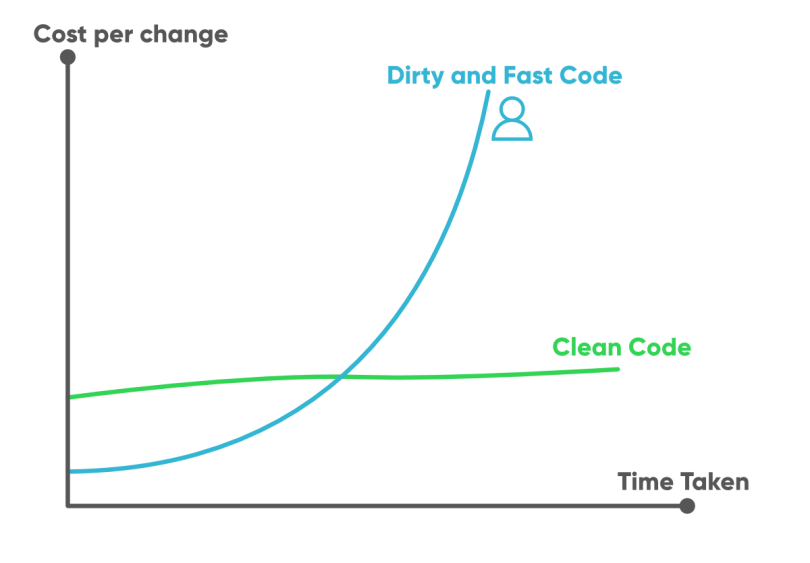
3. Return early
When writing your code try to avoid using too many nested if
statements. Nesting too deep will make your code less readable and a lot harder to understand for your colleagues. A technique you could use is returning early.
// Bad
class Product {
public function getPriceWithTax(): float
{
$priceWithTax = 0;
if (false === $this->downloadable) {
if (true === $this->taxable) {
if ($this->taxPercentage > 0) {
$priceWithTax = $this->price * (1 + ($this->taxPercentage / 100));
} else {
$priceWithTax = $this->price * 1.2;
}
} else {
$priceWithTax = $this->price;
}
}
return $priceWithTax;
}
}
// Good
class Product {
public function getPriceWithTax(): float
{
if (true === $this->downloadable) {
return 0;
}
if (false === $this->taxable) {
return $this->price;
}
if ($this->taxPercentage > 0) {
return $this->price * (1 + ($this->taxPercentage / 100));
}
return $this->price * 1.21;
}
}
Code language: PHP (php)
As you can see in the example, returning early makes your code a lot cleaner and easier to understand.
4. Function names should describe what they do
When naming your functions, you should give those functions descriptive names. Just like with variables, it makes the code more clear for your future self and your colleagues.
// Bad
class Cart
{
public function calculatePrice(array $products): float
{
// ...
}
}
// Good
class Cart
{
public function calculateTotalPriceWithTax(array $products): float
{
// ...
}
}
Code language: PHP (php)
The ‘Good’ method is much more explicit, and tells the developer reading the code much more about its functionality.
5. Don’t use flags as parameters
A method or function should do only one thing really well. This makes for great testable code. A flag parameter tells the developer a method does more than one thing based on the value of that flag. Let’s look at an example to see this in action.
// Bad
class Product
{
public function getPrice(bool $withTax): float
{
if (true === $withTax) {
return $this->price * (1 + ($this->taxPercentage / 100));
}
return $this->price;
}
}
// Good
class Product
{
public function getPriceWithoutTax(): float
{
return $this->price;
}
public function getPriceWithTax(): float
{
return $this->price * (1 + ($this->taxPercentage / 100));
}
}
Code language: PHP (php)
In the bad example, the getPrice
method has two tasks: returning the price without tax and returning the price with tax based on the boolean parameter. In the good example we’ve created an extra method to return the price with the tax. Now both methods have only one clear purpose as dictated by their name.
For more tips and tricks follow me on Twitter: @wcarabain
As always lovin content!
This is the way. ⭐ #cleancode
What is simple and good is doubly good, my aunt used to tell me.
great content as always 😉